In this tutorial, I will guide you through the step-by-step process of publishing .NET MAUI apps using Visual Studio Code and the ‘.NET MAUI – Archive / Publish tool’ extension by me. This extension simplifies the publishing process by providing a user-friendly interface within VS Code.
Without the help of an extension
You will need to dive into a terminal, and give out the following commands, based on which platform you want to build for.
# Android:
dotnet publish -f net8.0-android -c Release -p:AndroidKeyStore=true -p:AndroidSigningKeyStore={filename}.keystore -p:AndroidSigningKeyAlias={keyname} -p:AndroidSigningKeyPass={password} -p:AndroidSigningStorePass={password}
# iOS:
dotnet publish -f net8.0-ios -c Release -p:ArchiveOnBuild=true -p:RuntimeIdentifier=ios-arm64 -p:CodesignKey="Apple Distribution: John Smith (AY2GDE9QM7)" -p:CodesignProvision="MyMauiApp"
You can learn more, how to do it without my extension at:
https://learn.microsoft.com/en-us/dotnet/maui/ios/deployment/publish-cli?view=net-maui-8.0
and
https://learn.microsoft.com/en-us/dotnet/maui/android/deployment/publish-cli?view=net-maui-8.0
But don’t waste your time. I’ve put together a Visual Studio Code extension for you that will easily save you those unnecessary lines and allow you to publish from the UI.
Step 1: Install VS Code Extension
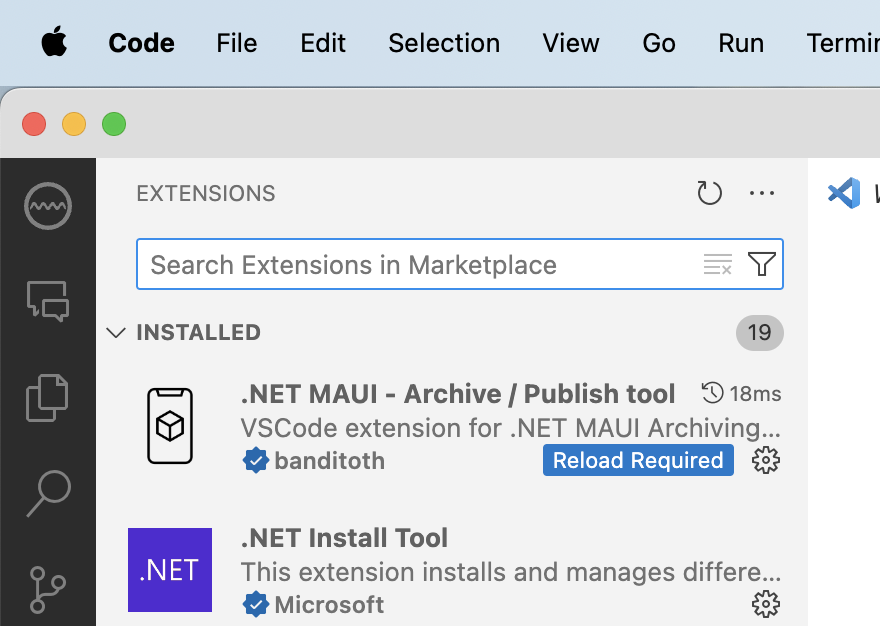
Visit the VS Code Marketplace and install the ‘.NET MAUI – Archive / Publish tool’ extension by ‘banditoth’.
You might be required to reload your VS Code instance.
Step 2: Open Your .NET MAUI Project
Launch Visual Studio Code and open your .NET MAUI project.
Step 3: Access the Command Palette
Press Ctrl+Shift+P
(Windows/Linux) or Cmd+Shift+P
(Mac) to open the command palette in Visual Studio Code.

Step 4: Choose Publish Android or Publish iOS
Type ‘MAUI Publish’ in the command palette. You will see two options: ‘MAUI: Publish Android’ and ‘MAUI: Publish iOS’. Select the one that corresponds to your target platform.
Publishing for Android:
The extension will prompt you to choose between a signed or non-signed binary. Select your preferred option.

Next, choose the package format – either apk
(Android Package) or aab
(Android App Bundle).

If signing is required (for a signed binary), the extension will list all installed keystore files. Choose the appropriate keystore.
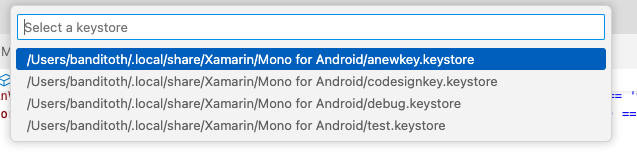
Enter the keystore password when prompted.

The extension will start the publishing process, and you’ll see progress information directly in the VS Code output.

Publishing for iOS:
Select ‘MAUI: Publish iOS’ from the command palette.
The extension will ask for the code signing identity. Choose the desired code signing identity from the available options.

Next, choose a provisioning profile to sign the app.

The extension will initiate the publishing process for iOS, displaying progress information in the VS Code output.
Conclusion
With the ‘.NET MAUI – Archive / Publish tool’ extension, publishing your .NET MAUI apps for Android and iOS becomes a straightforward process within Visual Studio Code. You no longer need to manually execute complex CLI commands; instead, you can leverage the extension’s user-friendly interface for a seamless publishing experience. Enjoy the convenience and efficiency of this simplified workflow for your .NET MAUI projects!
Support the project
You can find more information about how to contribute on this project at the project’s github page: https://github.com/banditoth/maui-archiver-vscode